Not the most efficient way of computing
As we very well know, there are a lot of ways to compute . There is even a blog entry in the Julia blog for that. Nevertheless, we decided for a different method (that is part of the introductory courses on JuliaAcademy).
A circle with radius has an area of
and the square that encases it
The ratio between the area of the circle and the area of the square is
and therefore we can define as
The same is true if we just take the first quadrant, so of the square as well as the circle. This simplification will make the code more compact and faster.
The above formula suggests that we can compute by a Monte Carlo Method. Actually this example is also included in the Wiki article and it comes with this nice gif.
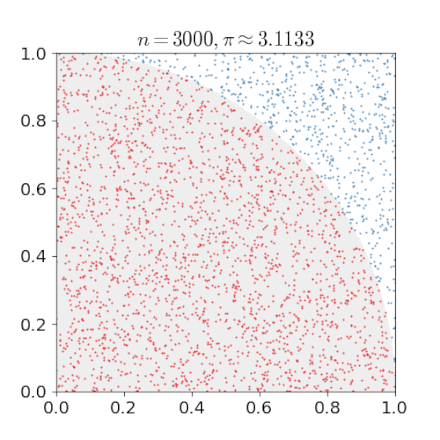
The algorithm therefore becomes:
For a given number of uniformly scattered points in the quadrant determine if these points are in the circle (distance less than 1) or not. We call the number of points in the circle .
Estimate by computing
Define a function that determines for a given integer .
Define a function that estimates for a given function and integer .
Test your code with different values for .
Help: The function rand()
will give you a uniformly scattered point in the interval .
function in_unit_circle(N::Integer)
M = 0
for i in 1:N
if (rand()^2 + rand()^2) < 1
M += 1
end
end
return M
end
function estimate_pi(f::Function, N::Integer)
return 4 * f(N) / N
end
function get_accuracy(f::Function, N::Integer)
return abs(
estimate_pi(f, N) - pi
)
end
N = 2^30
get_accuracy(in_unit_circle, N)
4.897092516031876e-5